Packages
每一个 go 程序都是有包(packages)组成的。
程序的执行是从main
包开始的。
如:
package main
import (
"fmt"
"math/rand"
)
func main() {
fmt.Println("My favorite number is", rand.Intn(10))
}
要使用包,只需使用 import
导入包的路径(paths of "fmt" and "math/rand"))即可
按照惯例,包名与导入路径的最后一个元素相同。
例如,“math/rand”包包含以语句package rand
开头的文件。
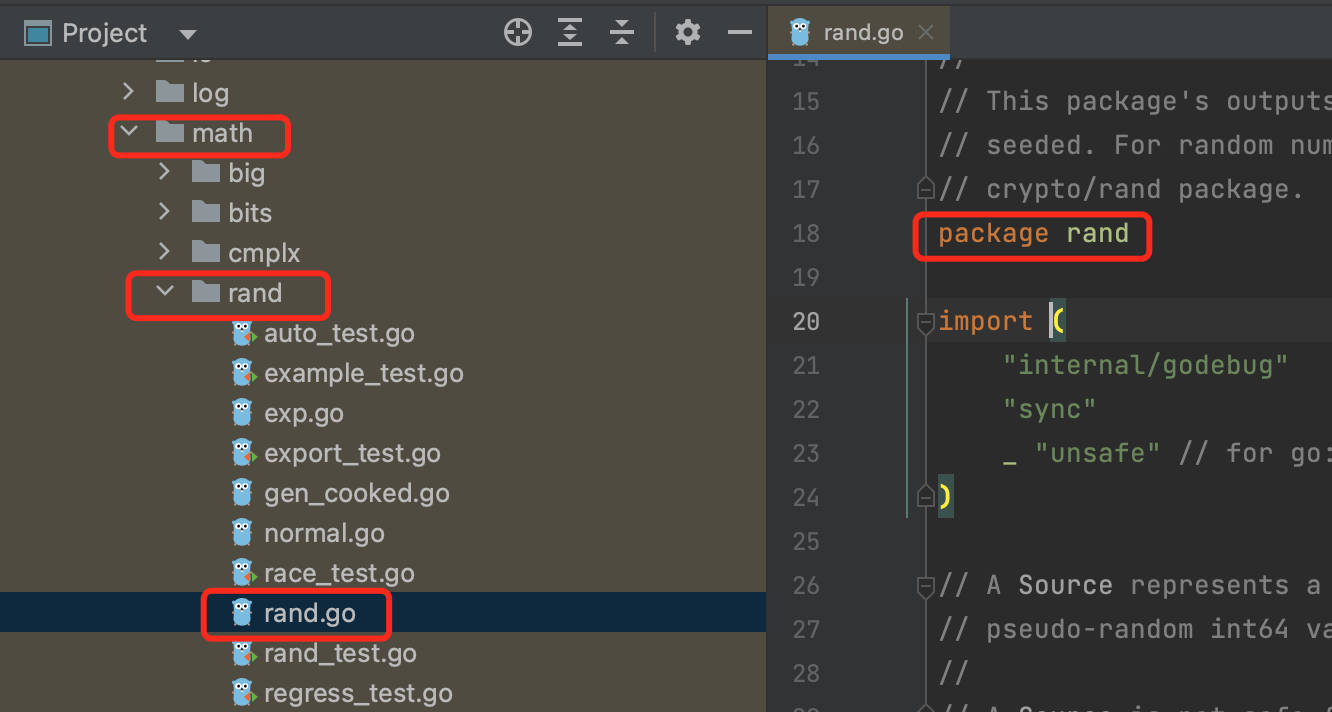
Imports
我们知道 import 可以导入包。
如果同时导入多个包,可以使用 因式分解导入语句(factored import statement),即包含在一个括号中。
import (
"fmt"
"math"
)
// 等价
import "fmt"
import "math"
推荐使用 the factored import statement.
为什么叫“factored” 呢? 是不是有点类似初中数学中的因式分解法。
This is the same as ab+ac=a(b+c), which is called factoring.
Exported names
在 Go 中,如果名称以大写字母开头,则导出名称。
导入包时,只能引用其导出的名称。
任何“未导出”的名称都不能从包外部访问。
package main
import (
"fmt"
"math"
)
func main() {
// 报错: undefined: math.pi
// To fix the error, rename math.pi to math.Pi and try it again.
fmt.Println(math.pi)
}
Functions
函数可以接受一个或多个参数,参数名称紧跟参数类型。
函数名称后面要指定函数返回值的类型。
// 定义一个函数,两个int类型的参数,返回值为int类型
func add(x int, y int) int {
return x + y
}
如果多个连续的命名参数,类型相同,可以省略除最后一个以外的所有类型。
func add(x, y int) int {
return x + y
}
函数也可以有多个返回值。
func swap(x, y string) (string, string) {
return y, x
}